Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial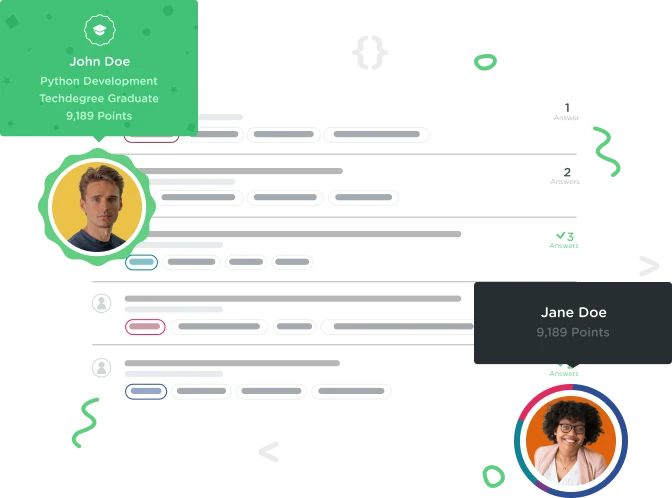

Julius Kucinskas
8,287 PointsWhy instance attributes don't always have to be passed in?
Why in classes instance attributes don't always have to be passed in ? When do they need to be passed in ? You pass it in or not you can still access it in all of the methods. ???
1 Answer
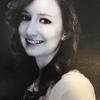
Megan Amendola
Treehouse TeacherHi Julius Kucinskas
Instance attributes don't have to be passed in when an instance is created. The reason why depends on the class you are creating, but essentially being able to pass in values gives you the ability to make each instance unique. Some attributes like maybe a name or age will probably be unique to each instance, while other attributes may not need to be unique.
Let's look at an example:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
self.sound = 'Woof woof!'
In the class above, I created 3 instance attributes, 2 of which are passed in when an instance is created, like this: jethro = Dog('Jethro', 2)
. The last attribute, self.sound
does not need to be passed in. All dog instances will be created with this sound.
If I need to change Jethro's sound, I can still do that by accessing the attribute and setting it equal to something else. jethro.sound = 'Boof!'
I can also still access all of these in a method:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
self.sound = 'Woof woof!'
def about(self):
return. f'{self.name} is {self.age} old and says "{self.sound}"'
Hopefully this helps. Let me know if you still have questions.