Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial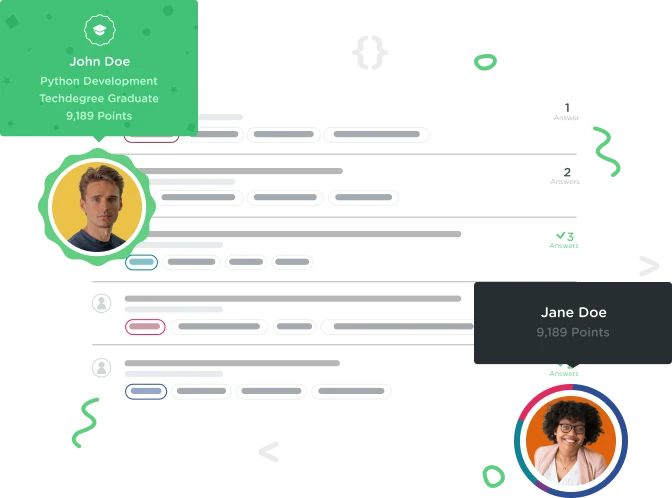

Derrick Hoff
9,385 PointsWhy is this saying my constructor isn't initializing properly?
can anyone help me I'm stuck on this problem I tried putting TongueLength in lower case and that didn't work either.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int TongueLength)
{
TongueLength = TongueLength;
}
}
}
1 Answer
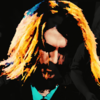
Justin Horner
Treehouse Guest TeacherHello Derrick,
The issue is that the compiler does not know the difference between your class member variable, TongueLength, and the identical parameter name in the Frog constructor.
When the compiler gets to this line it sets the value of TongueLength to itself.
TongueLength = TongueLength;
There are two ways to resolve this issue.
The first way is to change the parameter name in the constructor to begin with a lowercase "t". This is the naming convention for parameter names in C#. Remember that in C#, variables names are case sensitive so TongueLength is different than tongueLength.
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
The second way to resolve this issue is to scope the destination of the assignment to the class member so the compiler can differentiate between the class member and the local parameter variable in the constructor. Use the "this" keyword to reference the instance member of the class at runtime.
public Frog(int TongueLength)
{
this.TongueLength = TongueLength;
}
I hope this helps
Happy Coding :)