Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial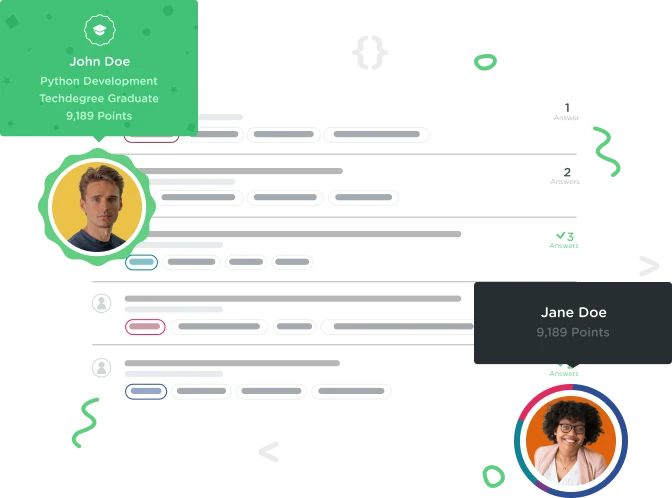
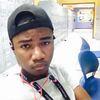
Christopher Downie
2,546 PointsWhy won't this be converted to upper case
Why is it not being converted to upper case, When i run the code it tells me that it inputted "abc" and expected to get back "ABC" but is is acually equal to "abc".
Can someone help me with this
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public String getDiscountCode() {
return discountCode;
}
public int getPriceInCents() {
return priceInCents;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = discountCode;
Order.normalizeDiscountCode(this.discountCode);
}
private static String normalizeDiscountCode (String normalizeCodeDis){
System.out.println(normalizeCodeDis);
normalizeCodeDis = normalizeCodeDis.toUpperCase();
return normalizeCodeDis; }
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
Joakim Mattsson
3,074 PointsJoakim Mattsson
3,074 PointsFirst of, the normalizeDiscountCode should not be static. Since you only call this method from within the Order class and the applyDiscoutCode method is not static. Therefore the normalize method don't need it.
Second, The first thing you are doing now is to apply the value to this.dicsountCode. After that, you put this.discountCode as a parameter in the normalize method.(this is not needed since you already have access to this data just by using this.discountCode from within the normalize method. BUT only if you delete static).
After this you printing the value and saying that the normalizeCodeDis = the uppercase version. (Correct). Then you returning the value. Nothing more.
The easy way of telling you whats wrong in your is that just put:
this.discountCode = Order.normalizeDiscountCode(this.discountCode);
But I wouldn't say its the correct way.
An easier version would look more like