Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial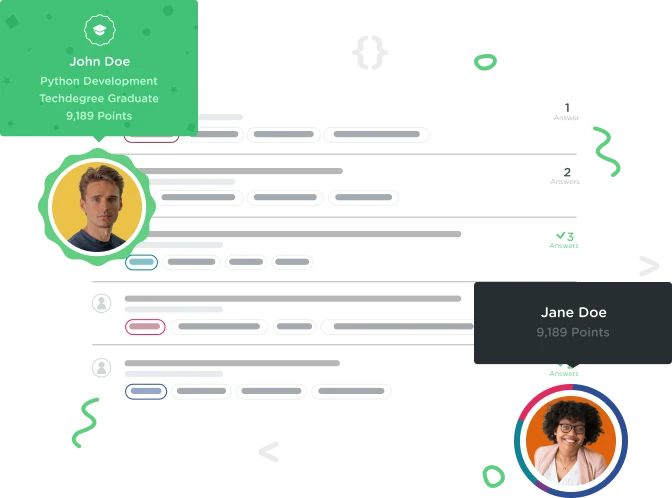

Jackie Wang
479 PointsYou probably already spotted the error in the drive method that accepts laps. You can drive way more laps than our batte
You probably already spotted the error in the drive method that accepts laps. You can drive way more laps than our battery can handle. Let's fix it.
Add logic to the drive method so that it throws an IllegalArgumentException if there aren't enough bars to support the laps request. Remember it takes 1 bar of energy to go around a lap.
I think it's good what's wrong with my program
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
int newAmount = lapsDriven + barCount;
if(newAmount < 0) {
throw new IllegalArgumentException();
}
}
}
3 Answers
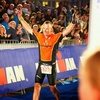
Steve Hunter
57,712 PointsHi Jackie,
There's a couple of ways of doing this depending on how you do your conditional test. However, what we're trying to achieve is a check as to whether the parameter passed in, laps
, is larger than the number of bars in the kart's possession, barCount
. So, if laps
is bigger tha barCount
, don't do any laps but do throw the exception. Alternatively, you can test if laps
is less than or equal to barCount
, if so, do the laps, else
throw the exception.
The first option is probably more elegant as it involves less code; I'll let you figure that one out, the second way would look something like this:
public void drive(int laps) {
if(laps <= barCount){
lapsDriven += laps;
barCount -= laps;
} else {
throw new IllegalArgumentException();
}
}
I hope that makes sense, if not, let me know and I'll try again!
Steve.
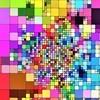
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyou don't need to make a new variable. you just need to compare laps with your remaining energy and if there's not enough energy, throw the error.

Jackie Wang
479 PointsSo which variable should I compare with

Jackie Wang
479 Pointsif(barCount < 0) {
throw new IllegalArgumentException();
}
if i do this it will say that Make sure you throw a new IllegalArgumentException if the requested amount of laps would make the battery less than zero.

Lawrence Babay
6,941 PointsYou definitely don't need a new variable here. All you have to do is compare the laps > barCount then throw the IllegalArgumentException.
Miguel Sánchez
10,365 PointsMiguel Sánchez
10,365 PointsThank you for your help!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!