Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player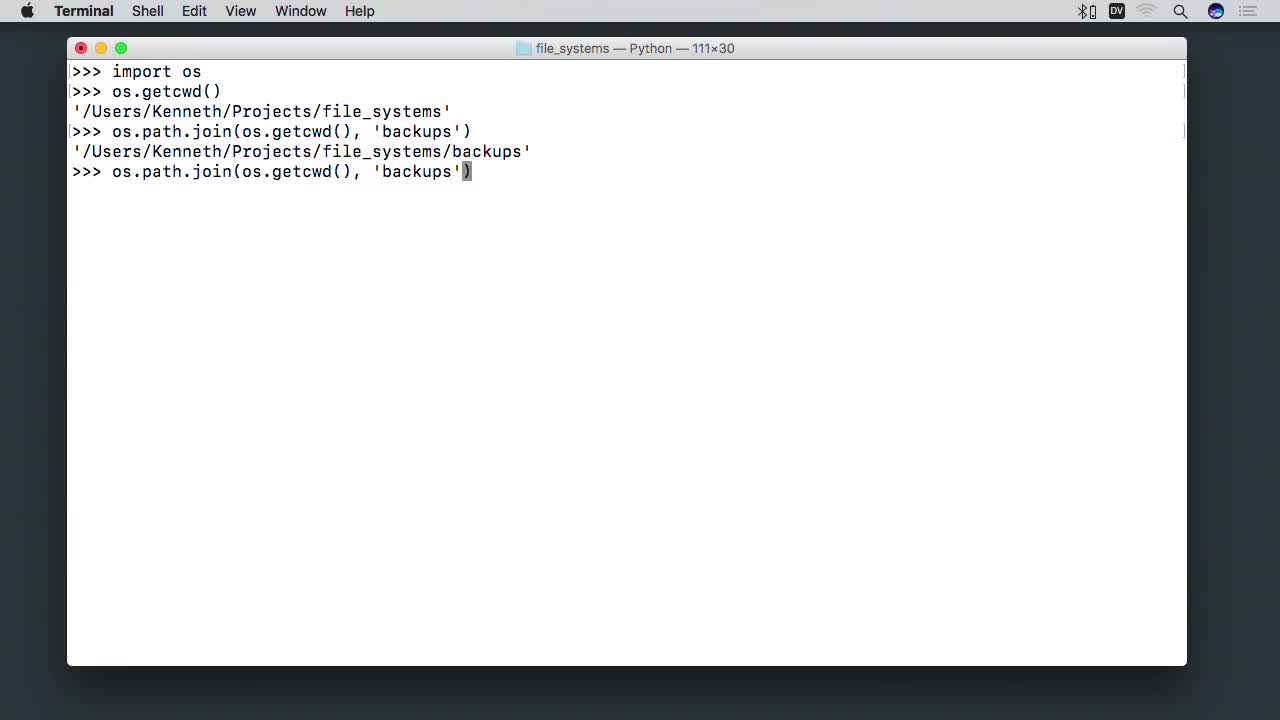
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Python has some great tools to simplify path creation. We'll learn how to automate this potentially tedious task.
Python Documentation
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
David Axelrod
36,073 Points2 Answers
-
PLUS
Hara Gopal K
Courses Plus Student 10,027 Points2 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
If you've spent any time creating paths
by hand, you know how annoying it can be.
0:00
Not only do you have to handle using
the right separators depending on which
0:04
operating system you're on, you also
have to deal with many other things.
0:06
Not all OSs care about upper or
lowercase letters.
0:09
Some softly enforce a character limit for
file and directory names.
0:12
Some require an extension for files, or
0:15
won't allow special characters
in directory names.
0:17
And then someone gives
you a relative path and
0:20
expects you to get to
the right place with it.
0:21
Users, am I right?
0:23
Well, you've probably heard
me say it before, and
0:24
you'll probably hear me say it again,
Python loves us and wants us to be happy.
0:26
And because of that,
we have the wonderful os.path.join tool.
0:29
Come on, let me show you how to use it.
0:33
All right, let's start right where we are.
0:34
I've imported os, I'm gonna do os.getcwd,
and I've got my file system's directory.
0:38
So, cool, we're used to this, right?
0:43
This is what we did in the last video.
0:45
Now, I wanna get the path for
the backups directory, though.
0:48
We could handwrite it, but that's boring.
0:51
Let's use this join thing
that I told you about.
0:53
It works kinda like when we join a list,
we don't have to provide the separator.
0:55
Python is smart enough to know
what kind of system we're on and
0:59
what the separator should be.
1:01
We pass in one or more paths, and
1:03
it will join them up correctly and
gave us back the new path.
1:04
So we can do os.path.join(os.getcwd(),
'backups').
1:07
And we get this new path.
1:16
We can do this with
multiple paths as well.
1:18
So we could add in, say,
a dot dot right there in the middle.
1:20
And now we would get
file_systems/../backups,
1:25
which would give us effectively,
/Users/Kenneth/Projects/backups,
1:28
cuz file_systems would
be removed due to that.
1:32
Now, that path doesn't exists, but
that's not the point of this function.
1:35
It doesn't care if the path is valid or
1:38
not, just that the path could
exist on the current systems.
1:40
Now, sometimes you need a bit
more power in your paths, though.
1:43
Python offers a whole module named pathlib
1:46
that provides a ton of power
when dealing with paths.
1:49
I won't go though all of it, but
let's play with it a little bit.
1:51
So first of all, we'll import pathlib.
1:53
And first, let's play with a peer path.
1:57
These act very similar to the paths
generated by os.path.join, except that
1:58
these are strings and these paths will
be objects that we can work with.
2:03
We'll start with a real path, though.
2:07
So say path=pathlib.PurePath(os.getcwd).
2:09
Okay, we know that's a real path.
2:17
That's the directory we're actually in.
2:18
So now let's say,
path2 = path / 'examples' / 'paths.txt.
2:19
And if we look at path2,
2:25
we get this PurePosixPath that
leads out to /examples/paths.txt.
2:27
Now what's up with all these
slashes that are in here, right?
2:33
That's weird.
2:38
That looks like division.
2:40
It's not division.
2:41
Paths from pathlib override
the division operator.
2:42
So instead of trying to do division,
it just joins together, but it's a path.
2:45
So path2 now represents
some imaginary location,
2:48
let's see what we can do with it.
2:51
So let's do path2.parts.
2:53
And we get a list of parts.
2:55
The parts attribute gives us a tuple
of all of the parts of the system.
2:58
I wanna point out here that
we only get one slash,
3:03
the one here at the beginning
which is the root of the system.
3:07
We can even check that.
3:10
We can say path2.root, and
it tells us that it's forward slash.
3:11
If we need to be able to access some of
the pieces that lead up to our final path,
3:14
we can use the parents attribute.
3:18
So, we'll do path2.parents and let's
just get the second item out of that.
3:19
So that we could [INAUDIBLE]
the first few directories there.
3:26
I'll leave this one for
you to play around with.
3:28
It's really neat, though,
so be sure that you do.
3:30
Two last attributes that I wanna show you.
3:32
If we need to get the final name,
whether it's a file name or
3:34
a directory name that the path points to,
we can use the name attribute.
3:37
So path2.name gives us paths.txt, and
3:40
we can use the suffix to
find out just the extension.
3:43
Now, I know I said that we wouldn't always
want to use the file's extension to tell
3:48
us what kinda file it is.
3:51
But you'll often find yourself wanting
to filter a list of files based on
3:52
the extension.
3:56
So it's handy to be able to find out.
3:57
I'll show you another way later on.
3:59
There is a concrete version of PeerPath
available too, known just as Path.
4:01
While it will work for
paths that don't currently exist,
4:05
it's meant to be used with
existing files and directories.
4:08
We may not use pathlib a lot in this
course, but it's very handy to know about.
4:10
And you'll find it makes working with
paths a lot easier for certain activities.
4:13
You can find a link to the full
documentation in the teacher's notes.
4:17
Now, though, let's see how to look
at what's inside of a directory.
4:21
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up